1. What is Python, and what are its key features?
Python is a high-level, interpreted programming language known for its simplicity and versatility. Let’s Explore Python Interview questions for freshers
Key Features: Interpreted, Object-Oriented, Dynamically Typed, Easy Syntax, Extensive Libraries, and Cross-Platform support.
2. Name a few Python data types?
Basic data types include:
- Integers (`int`)
- Floating-point numbers (`float`)
- Strings (`str`)
- Booleans (`bool`)
- Lists, Tuples, Dictionaries, and Sets for data structures.
3. How do you define a function in Python?
In Python, you define a function using the `def` keyword:
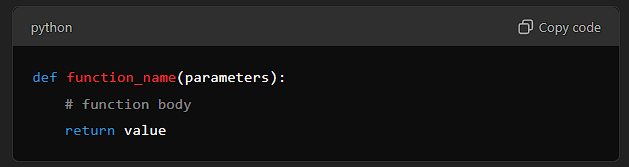
4. What are lists and tuples, and what’s the difference?
- List: Mutable, meaning elements can be modified. Defined with square brackets (`[]`).
- Tuple: Immutable, meaning elements cannot be modified once created. Defined with parentheses (`()`).
5. Explain Python’s ‘if-else’ statement.
In Python, the if-else
statement is used for making decisions.
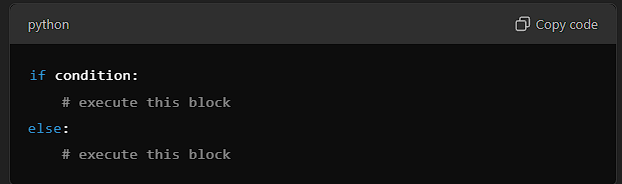
6. What is a Python dictionary?
A dictionary is a collection of key-value pairs, defined with curly braces (`{}`). Each key is unique and maps to a specific value.
7. What is the difference between ‘==’ and ‘is’ in Python?
- `==`checks for equality of values.
- `is`checks if two variables point to the same object (identity check).
8. How does Python handle memory management?
Python uses a private heap to manage memory, with automatic garbage collection to free unused memory.
9. What is the difference between `append()` and `extend()` in Python?
- `append()` adds a single element to the end of a list.
- `extend()` adds multiple elements from another list or iterable.
10. What are Python modules and packages?
- A module is a single Python file that can contain functions, classes, or variables.
- A package is a collection of modules organized in directories.
Conclusion
The Python interview questions listed here cover essential topics that are frequently asked in interviews, such as data types, functions, lists, and dictionaries. By practicing these Python interview questions, freshers can build a strong foundation and increase their chances of landing your dream job.